FileDownload
Download a file or data.
@solara.component
def FileDownload(
data: Union[str, bytes, BinaryIO, Callable[[], Union[str, bytes, BinaryIO]]],
filename: Optional[str] = None,
label: Optional[str] = None,
icon_name: Optional[str] = "mdi-cloud-download-outline",
close_file: bool = True,
mime_type: str = "application/octet-stream",
string_encoding: str = "utf-8",
children=[],
):
...
Simple usage
By default, if no children are provided, a button is created with the label "Download: {filename}".
import solara
data = "This is the content of the file"
@solara.component
def Page():
solara.FileDownload(data, filename="solara-download.txt", label="Download file")
Advanced usage
If children are provided, they are displayed instead of the button. The children can be any solara component, including a button, markdown text, or an image.
import solara
data = "This is the content of the file"
@solara.component
def Page():
with solara.FileDownload(data, "solara-download-2.txt"):
solara.Markdown("Any text, or even an image")
solara.Image("https://solara.dev/static/public/beach.jpeg", width="200px")
Any text, or even an image
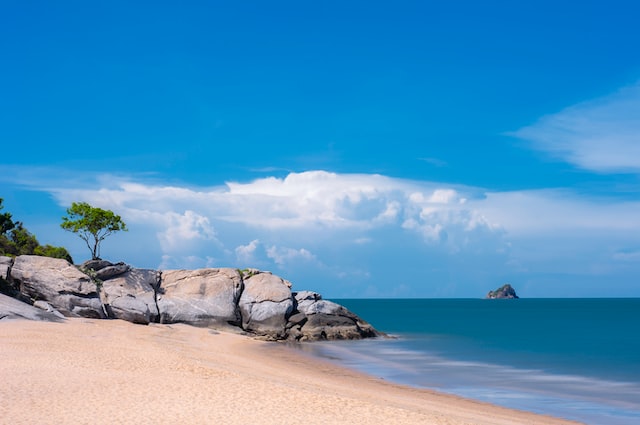
Custom button
If children are provided, they are displayed instead of the button. The children can be any solara component, including a button, markdown text, or an image.
import solara
data = "This is the content of the file"
@solara.component
def Page():
with solara.FileDownload(data, "solara-download-2.txt"):
solara.Button("Custom download button", icon_name="mdi-cloud-download-outline", color="primary")
Usage with file
A file object can be used as data. The file will be closed after downloading by default.
import solara
import pandas as pd
df = pd.DataFrame({"id": [1, 2, 3], "name": ["John", "Mary", "Bob"]})
@solara.component
def Page():
file_object = df.to_csv(index=False)
solara.FileDownload(file_object, "users.csv", mime_type="application/vnd.ms-excel")
If a file like object is used, we try to base the filename on the file object.
import solara
import solara.website.pages
import os
filename = os.path.dirname(solara.website.__file__) + "/public/beach.jpeg"
@solara.component
def Page():
# only open the file once by using use_memo
file_object = solara.use_memo(lambda: open(filename, "rb"), [])
# no filename is provided, but we can extract it from the file object
solara.FileDownload(file_object, mime_type="image/jpeg", close_file=False)
Lazy reading
Not only is the data lazily uploaded to the browser, but also the data is only read when the download is requested. This happens for files by default, but can also be used by passing in a callback function.
import solara
import time
@solara.component
def Page():
def get_data():
# I run in a thread, so I can do some heavy processing
time.sleep(3)
# I only get called when the download is requested
return "This is the content of the file"
solara.FileDownload(get_data, "solara-lazy-download.txt")
Arguments
data
: The data to download. Can be a string, bytes, or a file like object, or a function that returns one of these.filename
: The name of the file the user will see as default when downloading (default name is "solara-download.dat"). If a file object is provided, the filename will be extracted from the file object if possible.label
: The label of the button. If not provided, the label will be "Download: {filename}".icon_name
: The name of the icon to display on the button (Overview of available icons).close_file
: If a file object is provided, close the file after downloading (default True).mime_type
: The mime type of the file. If not provided, the mime type will be "application/octet-stream", For instance setting it to "application/vnd.ms-excel" will allow the user OS to directly open the file into Excel.string_encoding
: The encoding to use when converting a string to bytes (default "utf-8").
Note on file size
Note that the data will be kept in memory when downloading. If the file is large (>10 MB), and when using Solara server, we recommend using the static files directory instead.