Image
Displays an image from a URL, Path, numpy data, bytes or PIL image.
@solara.component
def Image(
image: Union[str, Path, "np.ndarray", bytes, "PIL.Image.Image"],
width: Optional[str] = None,
format="png",
classes: List[str] = [],
):
...
If passed as bytes, the image is assumed to be in the format specified by the format
argument.
Examples
Displaying an image from a Path
import solara
import solara.website
from pathlib import Path
image_path = Path(solara.website.__file__).parent / "public/beach.jpeg"
@solara.component
def Page():
solara.Image(image_path)
Live output
Displaying an image from a URL
import solara
image_url = "/static/public/beach.jpeg"
@solara.component
def Page():
solara.Image(image_url)
display(image_url)
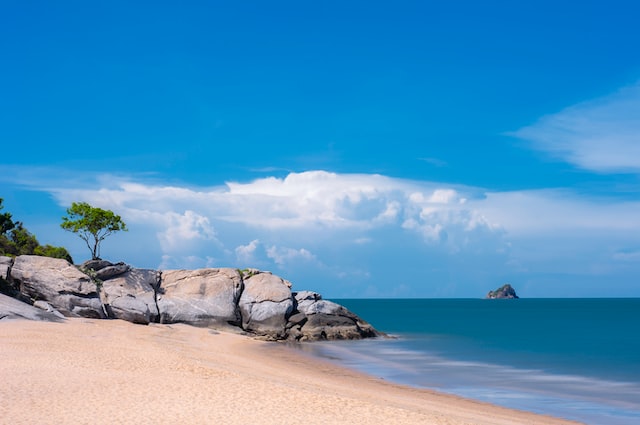
'/static/public/beach.jpeg'
Live output
Displaying an image from a numpy array
import solara
import solara.website
from pathlib import Path
import numpy as np
import PIL.Image
image_path = Path(solara.website.__file__).parent / "public/beach.jpeg"
image_ndarray = np.asarray(PIL.Image.open(image_path))
@solara.component
def Page():
solara.Image(image_ndarray)
display(image_ndarray)
array([[[ 1, 113, 199], [ 1, 113, 199], [ 1, 113, 199], ..., [ 0, 110, 199], [ 0, 110, 199], [ 0, 110, 199]], [[ 1, 113, 199], [ 1, 113, 199], [ 1, 113, 199], ..., [ 0, 110, 199], [ 0, 110, 199], [ 0, 110, 199]], [[ 1, 113, 199], [ 1, 113, 199], [ 1, 113, 199], ..., [ 0, 110, 199], [ 0, 110, 199], [ 0, 110, 199]], ..., [[237, 201, 189], [241, 205, 193], [240, 204, 190], ..., [130, 153, 194], [131, 154, 195], [131, 154, 195]], [[234, 198, 186], [239, 203, 191], [238, 202, 188], ..., [130, 153, 194], [131, 154, 195], [131, 154, 195]], [[240, 206, 196], [237, 203, 191], [232, 196, 182], ..., [130, 153, 194], [130, 153, 194], [130, 153, 194]]], dtype=uint8)
Live output
Displaying an image from bytes
import solara
import solara.website
from pathlib import Path
import PIL.Image
image_path = Path(solara.website.__file__).parent / "public/beach.jpeg"
image_bytes = image_path.read_bytes()
@solara.component
def Page():
solara.Image(image_bytes, format="jpeg")
display(image_bytes[:100])
b'\xff\xd8\xff\xe0\x00\x10JFIF\x00\x01\x01\x01\x00H\x00H\x00\x00\xff\xe2\x02\x1cICC_PROFILE\x00\x01\x01\x00\x00\x02\x0clcms\x02\x10\x00\x00mntrRGB XYZ \x07\xdc\x00\x01\x00\x19\x00\x03\x00)\x009acspAPPL\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'
Live output
Displaying an image from a PIL Image
import solara
import solara.website
from pathlib import Path
import PIL.Image
image_path = Path(solara.website.__file__).parent / "public/beach.jpeg"
image = PIL.Image.open(image_path)
@solara.component
def Page():
solara.Image(image)
Live output
Arguments
image
: URL, Path, numpy data, bytes or PIL Image.width
: Width of the image, by default (None) the image is displayed at its original size. Other options are: "100%" (to full its parent size), "100px" (to display it at 100 pixels wide), or any other CSS width value.format
: Format of the image, only used when image is of type bytes, or a PIL Image is passed.classes
: List of CSS classes to add to the image.